Flutter Tutorial: Tips, Tricks, and Best Practices for Developers
This Flutter tutorial is a comprehensive guide packed with tips, tricks, and best practices to help developers build high-performance, beautiful, and responsive mobile applications using the Flutter framework.
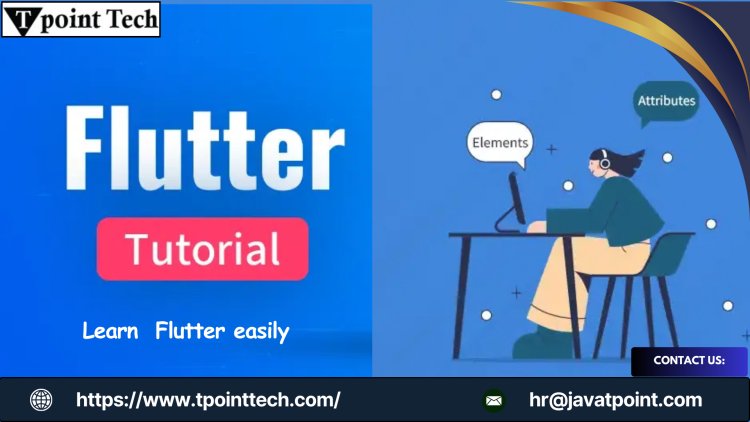
Flutter has revolutionized the way developers build mobile applications, providing a fast, efficient, and visually appealing framework for cross-platform development. Whether you are a Flutter tutorial for beginners or an experienced developer looking to optimize your workflow, mastering Flutter language requires understanding its best practices, tips, and tricks.
In this article, we will explore key techniques to enhance your Flutter development journey, ensuring smooth performance, maintainability, and a seamless user experience.
1. Understanding the Basics of Flutter Language
Before diving into advanced tips, it’s essential to understand the fundamentals of Flutter language. Flutter uses Dart, a modern, object-oriented programming language optimized for UI development.
Why Dart for Flutter?
- Fast execution: Dart compiles to native code for high-performance apps.
- Hot Reload: Allows instant UI updates without restarting the app.
- Rich Libraries: Offers powerful pre-built widgets and efficient asynchronous handling.
If you're just starting, check out the official Flutter documentation to get familiar with Dart’s syntax and concepts.
2. Optimize Your Flutter Code with Best Practices
Use the Right State Management Approach
Managing state efficiently is crucial for a smooth app experience. Flutter provides multiple state management solutions, including:
- Provider (Recommended for beginners) – Simple and powerful.
- Riverpod – A modern and improved version of Provider.
- Bloc (Business Logic Component) – Ideal for large-scale applications.
- GetX – Lightweight and easy to use.
Choose the right state management based on your project’s complexity and requirements.
Write Clean and Reusable Code
- Follow the DRY (Don't Repeat Yourself) principle.
- Break down UI elements into reusable widgets.
- Maintain a clear folder structure for better code organization.
For example, instead of defining the same button in multiple places, create a custom widget:
class CustomButton extends StatelessWidget {
final String text;
final VoidCallback onPressed;
const CustomButton({required this.text, required this.onPressed, Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: onPressed,
child: Text(text),
);
}
}
This approach enhances code maintainability and reduces duplication.
3. Improve App Performance in Flutter
Avoid Rebuilding Unnecessary Widgets
Flutter rebuilds widgets frequently, but excessive rebuilds can slow down performance. Use:
- const widgets to prevent unnecessary rebuilds.
- Consumer or Selector (if using Provider) to rebuild only necessary parts.
- shouldRebuild method in
ListView.builder
to optimize list performance.
Example of using const
to optimize UI:
const Text('Hello, Flutter!');
Use Lazy Loading for Lists and Images
- Implement ListView.builder for efficient scrolling in lists.
- Use cached_network_image package for optimized image loading:
CachedNetworkImage(
imageUrl: 'https://example.com/image.jpg',
placeholder: (context, url) => CircularProgressIndicator(),
errorWidget: (context, url, error) => Icon(Icons.error),
);
This reduces memory usage and improves app performance.
4. Debugging and Testing Tips
Use Flutter DevTools for Debugging
Flutter provides DevTools, a powerful debugging tool with features like:
- Widget inspector for UI debugging.
- Performance profiling to identify slow parts of your app.
- Network and memory monitoring.
To enable DevTools, run:
flutter pub global activate devtools
flutter run --debug
Write Unit and Widget Tests
Testing ensures app stability and prevents regressions. Flutter supports:
- Unit tests for business logic (
test
package). - Widget tests for UI components.
- Integration tests for full app flows.
Example of a simple widget test:
testWidgets('Check if button exists', (WidgetTester tester) async {
await tester.pumpWidget(MyApp());
expect(find.byType(ElevatedButton), findsOneWidget);
});
Writing tests helps catch bugs early and improves app reliability.
5. Best UI/UX Practices in Flutter
Use Material and Cupertino Widgets
Flutter provides two sets of UI components:
- Material Design (Android) –
ElevatedButton
,TextField
, etc. - Cupertino (iOS) –
CupertinoButton
,CupertinoTextField
, etc.
For cross-platform consistency, use the Platform
widget to switch between Material and Cupertino components based on the OS.
Implement Dark Mode
Dark mode improves usability and saves battery life. To add dark mode support:
MaterialApp(
themeMode: ThemeMode.system, // Follows system theme
theme: ThemeData.light(),
darkTheme: ThemeData.dark(),
);
Use Animations for a Smooth Experience
Animations enhance user engagement. Flutter provides built-in tools like:
Hero
for smooth page transitions.AnimatedContainer
for gradual changes in size, color, etc.Lottie
package for high-quality animations.
Example of using AnimatedContainer
:
AnimatedContainer(
duration: Duration(seconds: 1),
width: _isExpanded ? 200 : 100,
height: _isExpanded ? 200 : 100,
color: Colors.blue,
);
Animations make your app feel polished and responsive.
Conclusion
Mastering Flutter language involves not just learning the basics but also applying best practices to improve code quality, performance, and user experience. Whether you are following a Flutter tutorial for beginners or refining your skills as an experienced developer, these tips and tricks will help you build high-quality apps efficiently.
By implementing efficient state management, optimizing performance, following clean coding principles, and leveraging Flutter DevTools, you can create seamless and scalable applications.
Are you ready to take your Flutter development to the next level? Start implementing these best practices today and build amazing apps effortlessly! ????
What's Your Reaction?

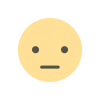




